Ice
Complete solution for distributed systems
A proven framework for network application development.
The Ice Framework
Provides everything you need to build networked applications
RPC Core
Ice is, at its core, an RPC framework that helps you build networked services with a high-level, easy-to-use API.
Powerful IDL
Describe the API of your networked services with Slice, an intuitive and flexible Interface Definition Language.
interface HealthWatcher {void update(string service,ServiceStatus status);}interface Health {ServiceStatus check(string service);void watch(string service,HealthWatcher* watcher);}
Stellar Services
Draw on Ice's collection of services for pub/sub, server deployment, and more.
IceStorm
IceGrid
Glacier2
DataStorm
Everywhere
Ice is available on all popular platforms, with mappings for 10 programming languages.
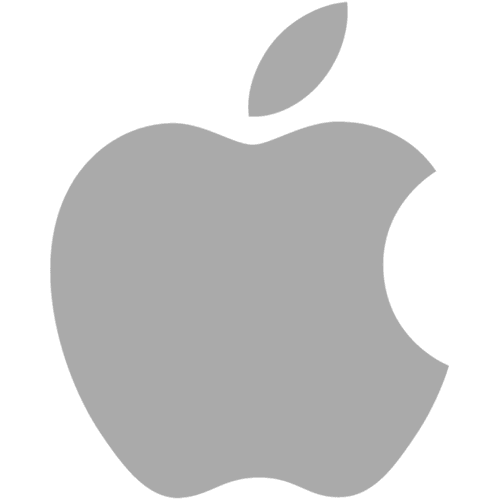
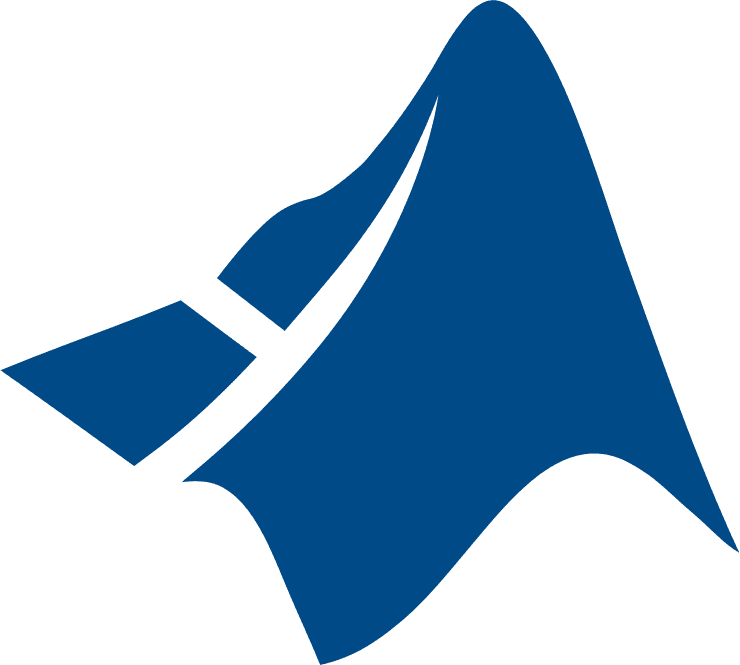
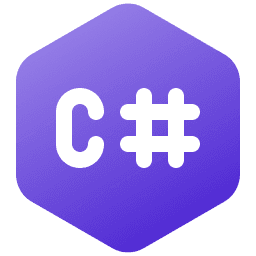
applications for over 5 years
RPC
Professional Grade
RPC Framework
Build your networked applications with the best tool for the job.
Efficient Communication
Reduce your CPU and bandwidth usage with Ice's efficient binary protocol.
Uniform API
Learn Ice in one programming language and your new skills immediately transfer to nine more languages, saving time in the development process.
Pick your transport
Choose from a variety of transports, including TCP, UDP, SSL, WebSocket and Bluetooth.
Slice
Strongly-Typed Network Calls Made Easy
Specify your network API in Slice, then let the Slice compiler generate the corresponding code in the language of your choice.
Familiar Syntax
The simple syntax with common constructs such as interface and class makes Slice easy to understand.
Binary Encoding
Enjoy lower bandwidth and CPU usage courtesy of Slice's compact and straightforward binary format.
module StarWars{enum JediRank { Youngling, Padawan, Knight, Master }class Jedi{string name;JediRank rank;optional(1) string padawanName;}sequence<Jedi> JediSeq;interface JediCouncil{JediSeq getCouncilMembers();void grantRank(string jediName, JediRank newRank);}}
Ready to get started?
It's time to jump in and start networking your software!